Alan AI Agentic Interface popups¶
To make the Alan AI agentic interface more noticeable and encourage users to interact with the Agentic Interface, you can show an Alan AI popup in the app.
The popup is a small window next to the Agentic Interface button that appears when a certain event is fired or action is performed. For example, you can choose to display the popup when the user launches the app or gives a specific voice or text command. For more details, see User events.
You can customize the popup in any way and add graphics and messages to it to attract the users’ attention.
Note
Alan AI agentic interface popups are currently supported on the Web platform.
To display a popup window, use the showPopup()
function in the script. In the example below, the popup window appears when the app is launched and the user clicks the Agentic Interface button for the first time:
onUserEvent((p, e) => {
console.info('EVENT', e.event);
if (e.event == 'firstClick') {
p.showPopup({
style:".card-img {width: 100%;margin-top: 0px;}.info-popup {background: #fff;max-width: 400px;height: 350px;width: 400px;max-height: 350px;box-shadow: 0px 1px 3px rgba(16, 39, 126, 0.2);overflow: hidden;border-radius: 10px;display: block;-webkit-box-orient: vertical;-webkit-box-direction: normal;-ms-flex-direction: column;flex-direction: column}.info-popup_header {padding: 0px;display: block;color: #000;font-size: 22px;font-weight: 700;text-align: center;background: #fff;background-repeat: no-repeat;background-position: center center;background-size: 100% 100%;}.info-popup_body {display: -webkit-box;display: -ms-flexbox;display: flex;-webkit-box-orient: vertical;-webkit-box-direction: normal;-ms-flex-direction: column;flex-direction: column;font-weight: 400;font-size: 20px;line-height: 18px;text-align: left;color: #000;padding: 9px 50px;height: 100px}.info-text {font-size: 14px;font-weight: 400;display: contents;margin: 0px;}",
html: '<div class="info-popup"><div class="info-popup_header"><img type="image/svg+xml" class="card-img" src="https://assets-global.website-files.com/64ec3fc5bb945b48c0a37b1c/6513eeb685e4c3f99bbd76b4_Customization_benefit2.webp"></div><div class="info-popup_body"><span class="info-text">Hi, I am Alan! <br/> Click the button to talk to me, <br/> ask questions and perform tasks</span></div></div>',
overlay: true,
buttonMarginInPopup: 15,
force: false,
});
}
});
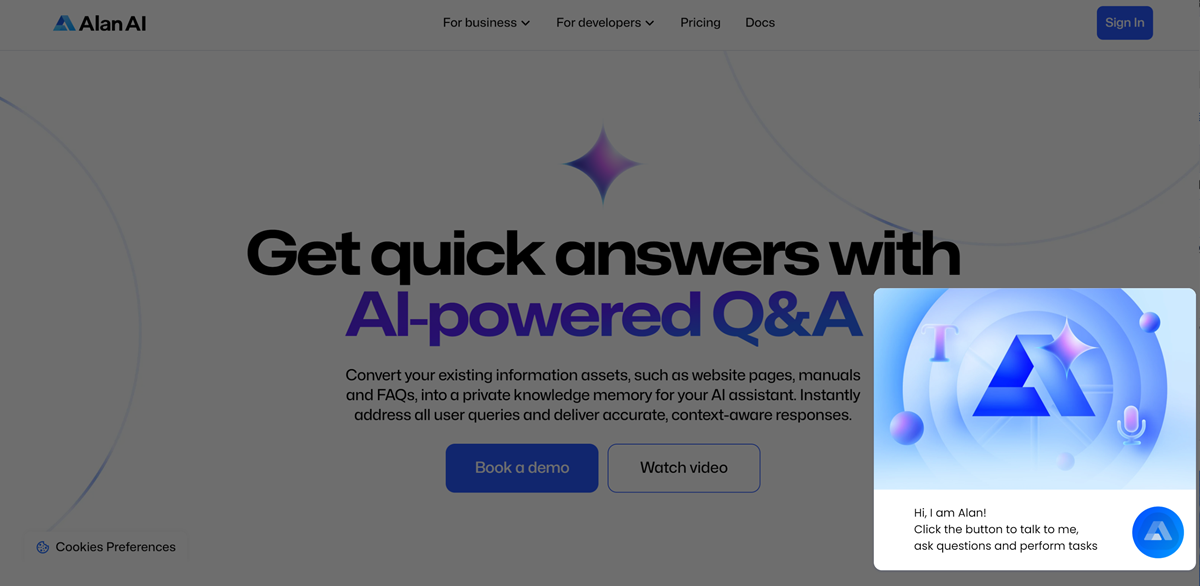
Popup parameters¶
The showPopup()
function takes the following parameters:
Name |
Type |
Description |
---|---|---|
|
HTML markup |
Contains the HTML markup of the popup window |
|
CSS markup |
Contains the styles of the popup window |
|
String |
Contains a path to a custom close popup icon |
|
Boolean |
Defines whether the overlay effect must be used ( |
|
Pixels |
Defines the margins for the Alan AI agentic interface in the popup window |
|
Boolean |
If |
|
Object |
Contains an input object to be applied to handlebar templates in the popup code. For details, see Handlebar templates in popups . |
Note
To ensure users provide microphone access to the Agentic Interface, you can also enable the overlay fade effect in the browser to make the microphone permission prompt more noticeable. For details, see Alan AI button parameters.
Popup editor¶
Alan AI Studio comes with the popup editor – a tool that allows you to preview UI widgets, such as buttons, cards and Alan AI agentic interface popups, and edit their code in a convenient way.
To open a UI widget in the popup editor:
In the code editor, to the left of
showPopup()
, click the edit icon.If you have several popups in the dialog script, in the left pane, select the widget you want to preview.
To prettify the code, at the top of the editor, click Format HTML, Format CSS and Format Params.
Edit the HTML, CSS code and update
params
data if needed.In the lower part of the editor, select the platform and device on which you want to preview the element. You can preview the element on a single device or choose to display all versions at once: browser, tablet and mobile.
To change the background, click the Device background color picker and choose the color.
To save the results, at the bottom of the left pane, click Save changes.
Note
To be rendered in the popup editor, the params
object should only contain numbers, strings, Boolean values or arrays/objects constructed with these values. If your params
object contains other types of values, the popup editor will fail to generate a real-time preview for your data.
You can edit the params data directly in the editor. Mind, however, that these changes will not be saved.