Sending commands to the app (React Native)¶
While interacting with the voice agent built with the Alan AI Platform, users can perform actions in the app with voice. For example, they can give commands to navigate to another screen, select an item in the list, enable or disable options. To achieve this, you need to send commands from the dialog script to the app and handle them on the app side.
In this tutorial, we will use a simple counter example to see how command handling works. The app users will be able to increment the counter with voice.
What you will learn¶
How to perform actions in the app with voice
How to send commands from the dialog script to the app
What you will need¶
You have completed all steps from the previous tutorial: Building a voice agent for a React Native app
You have set up the React Native environment and it is functioning properly. For details, see React Native documentation.
Step 1. Add the counter to the app¶
First, we need to add the counter to the app. Update the
App.js
file to the following:App.js¶import React, { useState } from 'react'; import { View, Text, Button, StyleSheet } from 'react-native'; const App = () => { const [count, setCount] = useState(0); incrementCount = () => { setCount(count + 1); } return ( <View style={styles.container}> <Text>You clicked {count} times</Text> <Button onPress={() => {this.incrementCount()}} title="Click me!" /> </View> ); }; // React Native Styles const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center' } }); export default App;
Add the Alan AI button as described in the previous tutorial:
Import
AlanView
Add the Alan AI button to the view
You can test it: run the app, tap the Alan AI button and give one of the commands available in the dialog script.
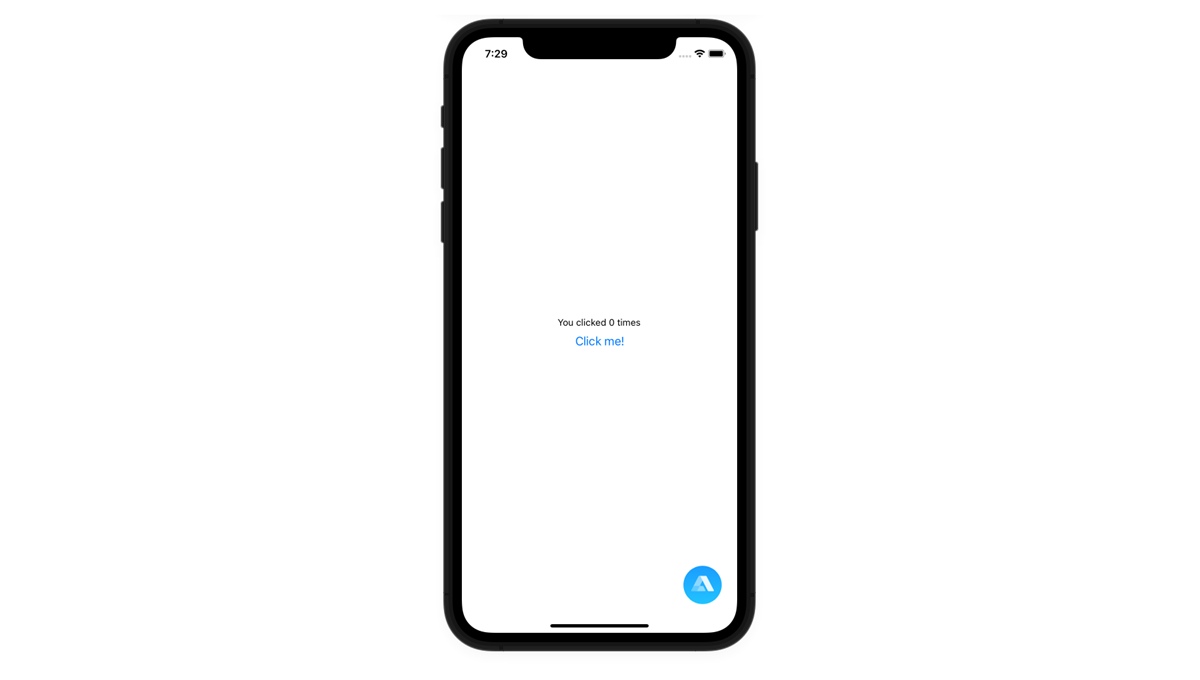
Step 2. Add a voice command to the script¶
To send a command to increment the counter to the app, add the following intent to the dialog script:
intent('Increment the counter', p => {
p.play('Incrementing the counter');
p.play({command:'setCounter'});
});
Here, we have two p.play()
functions:
One to play a response to the user
The other one to send the
setCounter
command to the client app. In this function, we have specified a JSON object with the name of the command to be sent.
You can try the command in the Debugging Chat. Notice that together with the answer, Alan AI now sends the command we have defined.
Step 3. Handle commands in the app¶
We need to handle this command on the app side. To do this, we will add the Alan AI’s onCommand handler to the app.
In the
App.js
file, add the import statement for the Alan AI’s events listener:App.js¶import { NativeEventEmitter, NativeModules } from 'react-native';
Create a new
NativeEventEmitter
object:App.js¶const App = () => { const { AlanEventEmitter } = NativeModules; const alanEventEmitter = new NativeEventEmitter(AlanEventEmitter); }
Update the following import statement to add the
useEffect
hook:App.js¶import { useState, useEffect } from 'react';
And add the
useEffect
hook to subscribe to the dialog script events:App.js¶const App = () => { useEffect(() => { alanEventEmitter.addListener('onCommand', (data) => { if (data.command == 'setCounter') { incrementCount(); } }) }, []); }
Now, when the app receives the setCounter
command, the incrementCount()
function will be invoked, and the counter will be incremented.
You can try it: in the app, tap the AI agent button and say: Increment the counter
.
What’s next?¶
Have a look at the next tutorial: Passing the app state to the dialog script.