Intent transforms¶
You can apply transforms to the intent()
function to modify how the Agentic Interface processes and outputs data when the intent is matched.
Example of use¶
Assume you have a JSON-formatted list of apartments. When the user asks: Show available apartments
, you want to display these appartments in an aesthetic format.
For this, you can do the following:
To the dialog script, add the following code:
Dialog script¶let data = { "apartments": [ { "name": "Modern Apartment in the City Center", "location": "123 Main Street, Cityville", "price": "$500,000", "bedrooms": 2, "bathrooms": 2, "image": "https://vmts.ch/wp-content/uploads/2017/06/v1.jpg", "url": "https://vmts.ch/en/portfolio/project-gasterzimmer/" }, { "name": "Suburban Family Home", "location": "456 Oak Avenue, Suburbia", "price": "$750,000", "bedrooms": 4, "bathrooms": 3, "image": "https://vmts.ch/wp-content/uploads/2017/05/7-2.jpg", "url": "https://vmts.ch/en/portfolio/project-niederteufen/" }, { "name": "Luxury Penthouse with Panoramic Views", "location": "789 Skyline Drive, Metropolis", "price": "$1,200,000", "bedrooms": 3, "bathrooms": 3, "image": "https://vmts.ch/wp-content/uploads/2017/06/Hallenbad_2-1.jpg", "url": "https://vmts.ch/en/portfolio/project-hallenbad/" } ] } intent("Show available apartments", async p => { p.play("Just a second..."); // Transform apartments data let a = await transforms.render({ input: data, query: 'Show available apartments' }); p.play(a); });
Here, when the user asks a question, the apartments data is passed to the
transforms.render()
function and formatted by its rules.In the Agentic Interface project, under Transforms, create the
render
transform with the following rules:In the Instruction field, provide general instructions on how to display apartments data:
Instruction¶The input contains the apartment description in JSON, the query contains sample questions, the result contains formatted text.
In the Examples section, add an example:
At the top of the Input field, select the data format: json. In the field below, enter sample JSON data describing apartments.
At the top of the Query field, select the data format: json. In the field below, enter the user query:
Show available apartments
.At the top of the Result field, select the data format: HTML. In the field below, add the HTML formatted text:
Transform example¶<div> <img src="https://vmts.ch/wp-content/uploads/2017/06/Hallenbad_2-1.jpg" alt="Luxury Penthouse with Panoramic Views"> <h4>Luxury Penthouse with Panoramic Views</h4> <p>Apartment details:</p> <ul> <li><b>Location:</b>789 Skyline Drive, Metropolis</li> <li><b>Price:</b> $1,200,000</li> <li><b>Bedrooms:</b> 3</li> <li><b>Bathrooms:</b> 3</li> </ul> <p><a href="https://vmts.ch/en/portfolio/project-hallenbad/">Learn more</a></p> </div> <br/>
Now, when you ask: Show available apartments
, the Agentic Interface will present apartments data as HTML.
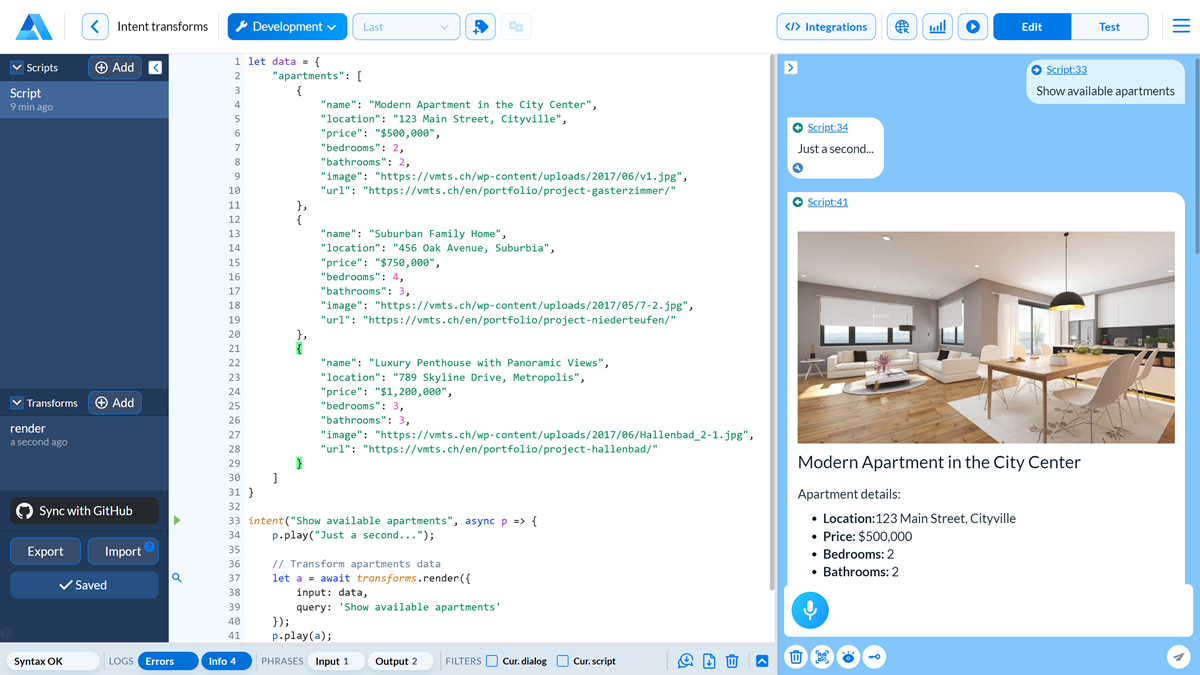