Intent matching¶
When the user says an utterance that closely resembles the invoking phrase of an intent, Alan AI evaluates the meaning of the phrase:
Automatic matching: if the user utterance is close enough in meaning to the invoking phrase, Alan AI will automatically match it to the intent and take the appropriate action.
Clarification needed: If the utterance is related but not an exact match, Alan AI will ask the user to clarify their request to ensure the right intent is triggered.
Cannot proceed: if the intent cannot be matched due to a lack of context or relevance, Alan AI will notify the user that it cannot proceed without more information.
Assume you have two intents that help users with delivery questions:
intent('What is the status of my delivery?', p => {
p.play('Let me check the status for you. Could you please provide your order number?');
});
intent('When will my order arrive?', p => {
p.play('Your order should arrive within 3-5 business days. Would you like more detailed tracking information?');
});
If the user asks about order delivery with phrases like:
When will my order arrive?
How long until my order gets here?
What’s the expected delivery date for my order?
Alan AI will automatically match this to the second intent and respond accordingly.
If the user asks a broader question, such as
Can you help with delivery questions?
, Alan AI will ask for clarification.If the user asks something unrelated, like
Can I sign up for a course?
, Alan AI will report an error.
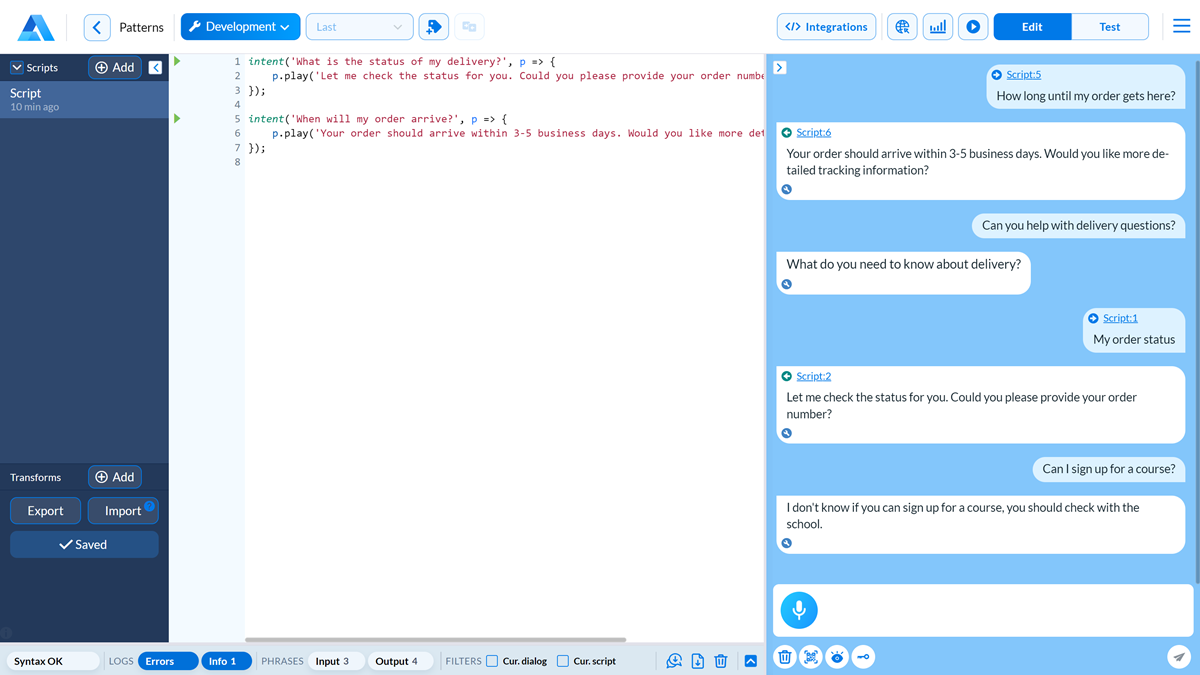